Howto Generate a Tileset and a Tilemap (CLI)
In this howtow, I will explain how to convert the image bellow to a GameBoy Tileset and a Tilemap that will be usable in a program to display the image on a real GameBoy (or on an emulator).
The image we want to display in our GameBoy program:
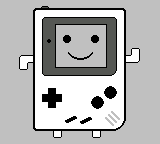
Generating a Tileset
First we have to convert the image into a GameBoy tileset… but what is a tileset ? To be displayed on a GameBoy, an image must be cut into 8x8 pixel pieces called tiles. A tileset is just… a set of tiles.
To generate the tileset, the command to use is the following:
img2gb tileset \
--output-c-file=tileset.c \
--output-header-file=tileset.h \
--output-image=tileset.png \
--deduplicate \
img.png
Note
For readability reason, I wrote the command on multiple lines using backslashes… you can of course write it on one line :)
Let’s explain the above command line:
tileset
tells img2gb that we whant to generates a tileset,--output-c-file=tileset.c
is the path of the output.c
file that will contain the data of the tiles,--output-header-file=tileset.h
is the path of the output.h
file that will contain information about the tileset (variable declaration and number of tiles),--output-image=tileset.png
is the path of the image that represents the tileset. It is useful to view what is in the.c
file in a more readable way. This image is also mandatory yo generate a tilemap.--deduplicate
avoids img2gb to include duplicate tiles (in our example, this is mandatory as the input image is composed of 360 tiles and the video memory of the GameBoy can contain only 255 tiles…),img.png
is the path of the input image.
Now let’s see the result of the above command:
-
// This file was generated by img2gb, DO NOT EDIT #include <types.h> const UINT8 TILESET[] = { 0xFF, 0x00, 0xFF, 0x00, 0xFF, 0x00, 0xFF, 0x00, // ... };
-
// This file was generated by img2gb, DO NOT EDIT #ifndef _TILESET_H #define _TILESET_H extern const UINT8 TILESET[]; #define TILESET_TILE_COUNT 97 #endif
-
Generating a Tilemap
The tilemap is table that tells the GameBoy where to display each tile to compose an image. To generate a tilemap, we first have to generate a tileset (see previous section).
To generate a tilemap you can use the following command:
img2gb tilemap \
--output-c-file=tilemap.c \
--output-header-file=tilemap.h \
tileset.png \
img.png
Once again, let’s explain this command:
--output-c-file=tileset.c
is the path of the output.c
file that will contain the data of the map,--output-header-file=tileset.h
is the path of the output.h
file that will contain information about the tilemap (variable declaration, with and height),tileset.png
is the path of the tileset image generated in the previous section,img.png
is the path of the image that will be mapped using the tileset.
Let’s take a look of the generated files:
-
// This file was generated by img2gb, DO NOT EDIT #include <types.h> const UINT8 TILEMAP[] = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x01, 0x02, // ... };
-
// This file was generated by img2gb, DO NOT EDIT #ifndef _TILEMAP_H #define _TILEMAP_H extern const UINT8 TILEMAP[]; #define TILEMAP_WIDTH 20 #define TILEMAP_HEIGHT 18 #endif
Using the Generated Code in a GameBoy Program
In this section I will only explain how to use the generated files in a GameBoy program that uses GBDK. I will not explain how to make a complete GameBoy program or how to compile it: that is not the purpose of this howto (but I will put some links at the end of this document).
Supposing you have a working folder that looks like this:
MyProject/
|
+-- main.c
|
+-- tilemap.c
|
+-- tilemap.h
|
+-- tileset.c
|
+-- tileset.h
Here is the minimal code to put in your main.c
file to use the tileset and
the tilemap:
#include <gb/gb.h>
#include "tileset.h"
#include "tilemap.h"
void main(void) {
// Load the tileset in the video memory of the GameBoy
set_bkg_data(0, TILESET_TILE_COUNT, TILESET);
// Load the tilemap in the Background layer of the GameBoy
set_bkg_tiles(0, 0, TILEMAP_WIDTH, TILEMAP_HEIGHT, TILEMAP);
// Make Background layer visible
SHOW_BKG;
}
That’s it :)
Links
Some article I wrote about GameBoy development (in French, but Google Translate should help):
Article (the first one of a series) explaining how to write, compile and execute a simple “Hello World” program on a GameBoy: https://blog.flozz.fr/2018/10/01/developpement-gameboy-1-hello-world/
A more complete article on the GameBoy graphics that use the same example that one of this document, but with more explanations: https://blog.flozz.fr/2018/11/19/developpement-gameboy-5-creer-des-tilesets/#convertir-des-images-avec-img2gb
Some examples of GameBoy programs that use img2gb:
The full code of this howto example (with a Makefile and a ROM): https://github.com/flozz/gameboy-examples/tree/master/05-graphics2
An example of the Background layer use: https://github.com/flozz/gameboy-examples/tree/master/06-graphics3-background
More links:
GameBoy Development Kit (GBDK): http://gbdk.sourceforge.net/